Adding Serverless to your Web Application
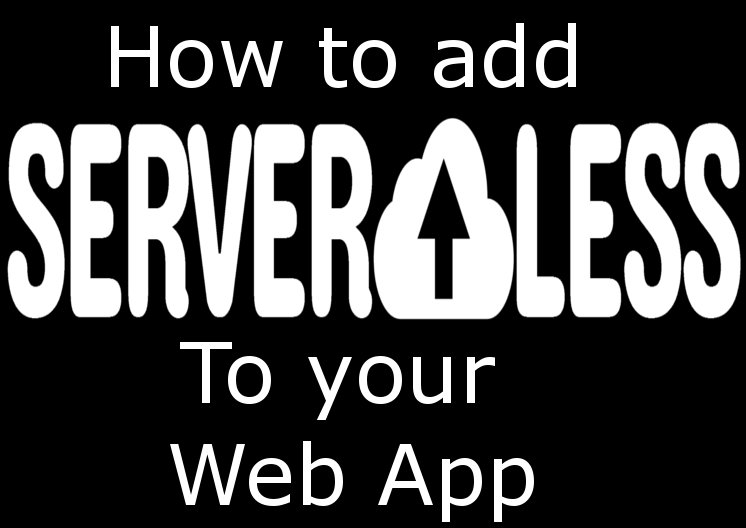
As you've probably heard, Serverless is the next big thing in the cloud industry.
Here's I'll go over some of the ways you can integrate Serverless technology in your existing web apps!
Serverless is essentially a system of Function-as-a-Service, where you have certain functions in the cloud, and you get billed by their usage & required computation.
This is a further abstraction from the actual Virtual Machines in common use today, and goes hand-in-hand with a Microservices approach to web development.
But enough about dictionary definitions, let's go to the good stuff:
Examples of integrating Serverless with your existing Web App
Reports
Database reports are a pretty common request, often by marketing/business departments to get a better understanding of your users.This is more common in early-stage applications, before you connect them to proper analytics tools.
Using Serverless, it's quite simple to have a function that runs a report on the database.
We'll use AWS Lambda in this example:
- Go to Lambda on your AWS Dashboard:
- Choose "Author from scratch":
- In your Lambda function, add the following code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
'use strict'; module.exports.runReport = (event, context, callback) => { const response = { statusCode: 200, body: { message: 'Result: ', input: event, }, }; const mysql = require('mysql'); const connection = mysql.createConnection({ host: 'localhost', user: 'user', password: 'password', database: 'database', port: 'port' //if you remove this line port defaults to 3306 }); connection.connect(); connection.query('SELECT * FROM users', function(err, results) { if (err) throw err; response.body.message += JSON.stringify(results); response.body = JSON.stringify(response.body); callback(null, response); }) connection.end(); };
- As an easier alternative, get the entire Serverless Boilerplate here
Admin Panel
In most web applications there's some sort of an Admin Panel.Ideally, the admin panel should be a separate application, to limit potential systemic weaknesses.
Using Serverless, we're able to take advantage of some of the most secure systems available - IAM permissions.
Examples of cool things you could do to further secure your Admin Panel:
- Restrict Admin functions permissions - Since Lambda functions have their own IAM roles, you can have very fine-grained permission settings. A great example of this is with DynamoDB.
You can literally give your function access to specific tables with a custom IAM policy:Or another great example where a function can only write items to the table without reading any:1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
{ "Version": "2012-10-17", "Statement": [ { "Sid": "Stmt1509470869566", "Action": [ "dynamodb:BatchGetItem", "dynamodb:DescribeTable", "dynamodb:GetItem", "dynamodb:GetRecords", "dynamodb:ListTables", "dynamodb:Query", "dynamodb:Scan" ], "Effect": "Allow", "Resource": "arn:aws:dynamodb:YOUR-REGION:YOUR-AWS-ID:table/TABLE-NAME" } ] }
1 2 3 4 5 6 7 8 9 10 11 12 13
{ "Version": "2012-10-17", "Statement": [ { "Sid": "Stmt1509470869566", "Action": [ "dynamodb:PutItem" ], "Effect": "Allow", "Resource": "arn:aws:dynamodb:YOUR-REGION:YOUR-AWS-ID:table/TABLE-NAME" } ] }
- Combine API Gateway with Cognito User Pools for authentication - This is quite complex, but this guide has a pretty good walkthrough. In the following days (probably weeks) I'll try to add this to the boilerplates repository.
- Write the entire Admin Panel on Serverless - If your app doesn't have a fully fledged Admin Panel yet (and you understood the last 2 items on the list), This could offer both a high level of security as well as a great way to integrate Serverless into an important but not-yet-customer-ready parts of your system.
Cron Jobs
Most applications have some form of Cron Jobs, but more often than not,there's a good chance that you don't have a lot of logging/analytics for your Cron Jobs.
With CloudWatch you can schedule functions to run every minute/hour/day/week, just like a normal Cron Job.
Combine that with CloudWatch Alarms, and you have both a logging system and an alarm system in case something goes wrong.
These were just a few examples of how you could integrate Serverless into your web app right away.
In the future, I'll try to work on the boilerplate repository, to add more cases.
Posted in
Technology